Everything about java.lang.ArrayIndexOutOfBoundsException
Introduction
The ArrayIndexOutOfBoundsException
is thrown when we try to access an array with an illegal index.
In this guide we will look at the exception in detail along will an exhaustive list of scenarios which can cause this exception and ways to resolve them.
What is ArrayIndexOutOfBoundsException?
An illegal index is any index other than 0
to array.length - 1
.
For example, if you have an array of size 5, a valid index would be anything between 0
and 4
.
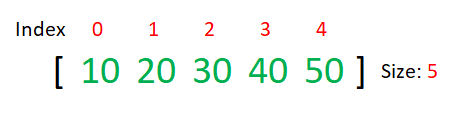
Anything less than 0
or greater than 4
would be an illegal index and would throw ArrayIndexOutOfBoundsException
.
There are several scenarios which can cause this exception. We will see each one with examples and solutions in this guide.
Common Causes and Solutions
1. Incorrect index check in loops
Cause: One of the most common scenario of ArrayIndexOutOfBoundsException
is while looping through an array based on its index.
Example of the issue:
int[] array = {10, 20, 30, 40, 50};
for(int i = 0; i <= array.length; i++) {
System.out.println(array[i]);
}
Solution:
int[] array = {10, 20, 30, 40, 50};
for(int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
Detailed Explanation: In this example, the i <= array.length
check is the cause of our exception. This mean the for loop will run from index 0
till the index that equals length of the array which is 5
. But Since the array index ends at 4
, this will cause ArrayIndexOutOfBoundsException
.
To resolve the issue we changed the condition to i < array.length
so that the for loop correctly loops through the indexes 0
to 4
.
2. Getting array value without checking length
Cause: Before getting value from an array, we need to check if the value is actually available or else we will get ArrayIndexOutOfBoundsException
.
Example of the issue:
int[] array = someMethodThatRetunsArray(); //Suppose this returns empty array
System.out.println(array[0]); //This throws exception
Solution:
int[] array = someMethodThatRetunsArray(); //Suppose this returns empty array
if(array.length > 0) { //This returns false so we avoid exception
System.out.println(array[0]);
}
Detailed Explanation: In this example, the someMethodThatRetunsArray()
returns an empty array so trying to read any value (in our case array[0]
) from the array will result in ArrayIndexOutOfBoundsException
.
This is true for arrays which have values as well. Let's say you have an array of size 2
and you try to read array[2]
from it, it will throw exception.
To resolve the issue always check if the array has necessary number of values to support the index we want to get. If we want to do array[2]
we need to have an array of size at least 3
.
3. Not checking length of String.split() result
Cause: The String.split()
method returns an array and if we try to access an index which is not in the array it causes ArrayIndexOutOfBoundsException
.
Example of the issue:
String fullName = "Mike Wazowski";
String lastName = fullName.split(" ")[1]; //Works fine as fullName has a last name separated by empty space
fullName = "Mike";
lastName = fullName.split(" ")[1]; //Throws exception as there is no empty space and last name
Solution:
String fullName = "Mike";
String[] nameSplitted = fullName.split(" ");
if(nameSplitted.length > 1) {
String lastName = nameSplitted[1];
}
Detailed Explanation: In this example, we are splitting the fullName
by space character. But if there is no empty space, the output array of split()
operation has only one element (the entire fullName
string). So trying to get index 1
from it results in ArrayIndexOutOfBoundsException
.
To resolve the issue, you can either check if the input string has the delimiter (space in our example), or even better check the split output array length as shown in the solution.
4. String.split() with regex special characters
Cause: String.split()
has a special case while using regex characters which can cause ArrayIndexOutOfBoundsException
.
Example of the issues:
String domain = "google.com";
String tld = domain.split(".")[1]; //Throws exception
Solution:
String domain = "google.com";
String tld = domain.split("\\.")[1];
Detailed Explanation: Split considers regex characters like .
, ^
, etc as special characters and does not split the string with the literal character. So although the domain has a dot character, it didn't split the string with it hence the resulting array has only one element. So trying to get index 1
from it results in ArrayIndexOutOfBoundsException
.
To resolve the issue, we have to escape such special characters by \\
.
But what if the delimiter itself is dynamic? You can use the Pattern.quote()
method as shown below to escape all regex characters.
String domain = "google.com";
String tld = domain.split(Pattern.quote("."))[1];
5. Setting element to array in invalid index
Cause: Java arrays are created with a fixed size. If we try to set an element with an index more than the available capacity, it will cause ArrayIndexOutOfBoundsException
.
Example of the issues:
int[] array = new int[0];
array[0] = 10;
Solution:
//Solution 1: Initialize array with necessary capacity
int[] array = new int[1];
array[0] = 10;
//Solution 2: Check array length before setting value to an index
int[] array = new int[0];
if(array.length > 0) {
array[0] = 10;
}
Detailed Explanation: Arrays have fixed size provided during instantiation. In our example, we created an array of size zero, which means it can't hold any element. So trying to add an element in index 0
result in ArrayIndexOutOfBoundsException
.
To resolve the issue, we can either set the array size to necessary size to hold all our values or we can check array length before setting a value to an index.
6. Accessing varargs with invalid index
Cause: Varargs with syntax like String[]
or String...
can be used to accept dynamic number of arguments in a method. A classic example of this is the String[] args
in java main
method which is used to pass command-line arguments. If we try to get an element by index from vararg which is not actually present, it will cause ArrayIndexOutOfBoundsException
.
Example of the issue:
public static void main(String[] args) {
System.out.println(args[0]);
}
Solution:
public static void main(String[] args) {
if(args.length > 0) {
System.out.println(args[0]);
}
}
Detailed Explanation: In the example, if no command-line argument is passed while executing the Java program, it causes ArrayIndexOutOfBoundsException
as there is no element in the array hence args[0]
fails.
To resolve the issue, we need to check the varargs length before trying to access the value to an index.
7. Getting and setting invalid index in Arrays.asList()
Cause: Arrays.asList()
creates a fixed size List
which pretty much means it have similar restrictions like an array. If you try to get or set value from/to a list created with Arrays.asList()
with an invalid index, it will cause ArrayIndexOutOfBoundsException
.
Example of the issue:
List<Integer> numbersList = Arrays.asList(10);
numbersList.set(1, 20); //Causes ArrayIndexOutOfBoundsException
numbersList.get(1); //Causes ArrayIndexOutOfBoundsException
Solution:
List<Integer> numbersList = Arrays.asList(10);
if(numbersList.size() > 1) {
numbersList.set(1, 20);
numbersList.get(1);
}
Detailed Explanation: In the example, the list is created with an array/vararg of one element, so the only valid index in the list is 0
. So when we try to get()
or set()
an element to index 1
, it causes ArrayIndexOutOfBoundsException
.
To resolve the issue, we need to check the list size before trying to access the value to an index.
Note: If we created an array list instead with new ArrayList()
and try to get()
or set()
to an invalid index, it causes IndexOutOfBoundsException
instead of ArrayIndexOutOfBoundsException
.
Additional Considerations
Although there are several scenarios, the underlying issue is very similar. So below points can be a good general guide to avoid ArrayIndexOutOfBoundsException
.
- Be aware of index/size difference: Indexes start from
0
in Java so be aware that for an array of sizeN
, valid index will be from0
toN-1
and not1
toN
. - Check for array length: Check array length before doing an index based operation will ensure we don't face the exception.
- Use enhanced for loop when appropriate: If you don't need the index value, you can just use the enhanced for loop to loop through the values instead of index based operations.
int[] array = {10, 20, 30};
for(int value : array) {
System.out.println(value);
}
Conclusion
ArrayIndexOutOfBoundsException
is one of the most common exception in Java. I hope this guide was helpful in identifying and resolving the issue.